Building Scalable and SEO-Friendly Web Apps with Server-Side Rendering in React or Vue.js
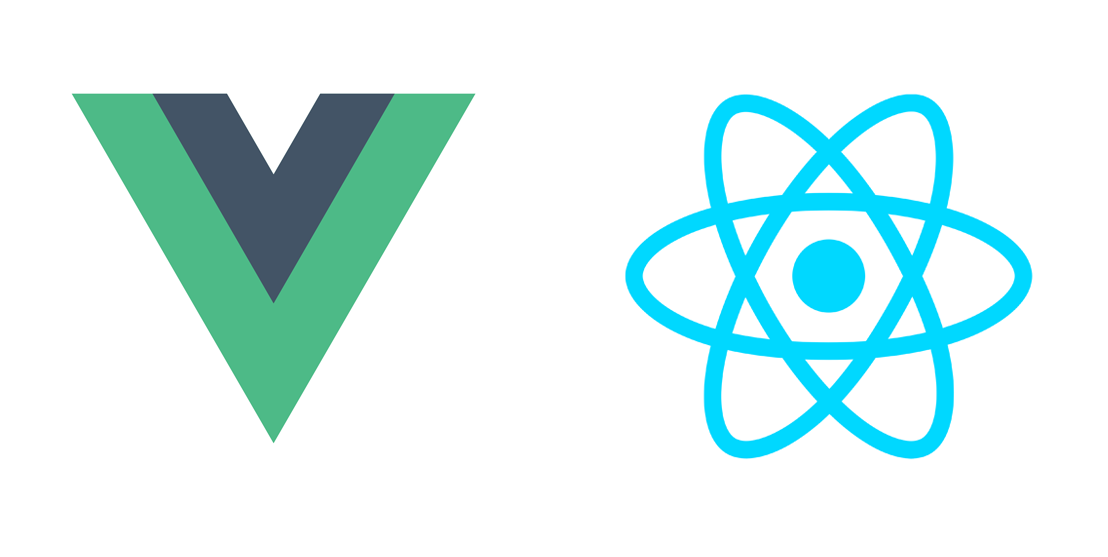
Building a modern web application involves a wide range of technologies and techniques, but at its core it typically involves a front-end application running in the user’s web browser and a back-end application running on a server. In this tutorial, we will focus on building the front-end of the application using a popular JavaScript framework such as React or Vue.js.
One of the key decisions when building a web application is whether to use a server-rendered or a single-page application (SPA) architecture. A server-rendered application is one in which the server generates the HTML for each page of the application, while a SPA is a client-side application that loads a single HTML page and then updates the page dynamically as the user interacts with the application. Both approaches have their advantages and disadvantages, and the choice will depend on the specific requirements of your application.
In this tutorial, we will focus on building a server-rendered application using a JavaScript framework such as React or Vue.js. Server-rendered applications are often a good choice for applications that require search engine optimization (SEO) or that have complex user interfaces with many interacting components. They can also be a good choice for applications that need to work well on devices with limited processing power or network connectivity.
To build a server-rendered application using a JavaScript framework, we will need to set up a few things:
- A development environment: This will typically include a text editor, a command-line interface (CLI), and a package manager such as npm or yarn.
- A build system: This will typically be a tool such as webpack or Parcel that can transpile and bundle our JavaScript code into a format that can be run in a web browser.
- A server-side rendering library: This will be a library such as ReactDOMServer or vue-server-renderer that can generate the HTML for our application on the server.
- A server: This could be a simple Node.js server using a library such as Express, or it could be a more complex server-side application using a framework such as Rails or Django.
Once we have these things set up, we can start building our application. A typical server-rendered application will have the following structure:
- A set of components: These will be the building blocks of our user interface, and they will typically be written using a declarative syntax such as JSX in React or template strings in Vue.js.
- A router: This will be responsible for mapping the current URL to the appropriate set of components to render.
- A server: This will be responsible for rendering the HTML for each page of the application and serving it to the user’s web browser.
To build our application, we will follow these steps:
- Set up our development environment and build system.
- Write our components using a declarative syntax such as JSX or template strings.
- Set up our router to map URLs to components.
- Write a server-side rendering function that generates the HTML for each page of the application using our components and router.
- Set up a server to serve the generated HTML to the user’s web browser.
That’s a high-level overview of the process of building a modern, server-rendered web application using a JavaScript framework. In a real-world application, there will be many additional details and considerations, but this should give you a good starting point.
One important aspect of building a server-rendered application is ensuring that the application can be easily built and deployed. This usually involves setting up a continuous integration (CI) and deployment (CD) pipeline that can automatically build and deploy the application whenever new code is pushed to a version control system such as Git. There are many tools available for setting up CI/CD pipelines, such as Jenkins, Travis CI, and GitHub Actions.
Another important aspect of building a server-rendered application is ensuring that the application is performant and scalable. This usually involves optimizing the performance of the server-side rendering process, caching rendered pages, and optimizing the performance of the client-side application. There are many tools and techniques available for optimizing the performance of web applications, such as code splitting, tree shaking, and lazy loading.
Here is an example of building a simple server-rendered application using React and Express:
First, we will set up our development environment and build system. This will typically involve installing Node.js and a package manager such as npm or yarn, and setting up a text editor such as Visual Studio Code.
- Install Node.js: Node.js is a JavaScript runtime that allows you to run JavaScript code on the server side. To install Node.js, visit the Node.js website (https://nodejs.org/) and follow the instructions for your operating system.
- Install a package manager: A package manager is a tool that allows you to install and manage third-party libraries and tools that your application depends on. The most popular package managers for Node.js are npm (which is installed with Node.js) and yarn. To install yarn, visit the yarn website (https://yarnpkg.com/) and follow the instructions for your operating system.
- Set up a text editor: A text editor is a program that allows you to write and edit code. There are many options available, including popular choices such as Visual Studio Code, Sublime Text, and Atom. To install Visual Studio Code, visit the Visual Studio Code website (https://code.visualstudio.com/) and follow the instructions for your operating system.
- Create a new project directory: Open a terminal or command prompt and navigate to the directory where you want to create your new project. Then, use the mkdir command to create a new directory for your project:
mkdir my-app
- Initialize a new npm project: Navigate to your new project directory and use the npm init command to initialize a new npm project:
cd my-app npm init -y
The -y
flag will accept the default values for the prompts, creating a package.json
file with the default settings. You can also omit the -y
flag and answer the prompts to customize the settings for your project.
That’s it! You have now set up your development environment and build system and created a new project directory and npm project. In the next steps, you will install the dependencies you need to build your application and start writing code.
Now we can install the dependencies we need to build our application, including React, ReactDOM, and Express:
npm install react react-dom express
Next, we will create a file called server.js in the root of our project directory and write the code for our server. This code will use the Express library to create a simple server that listens for HTTP requests and serves the same HTML page for all requests:
const express = require('express'); const app = express(); app.get('*', (req, res) => { res.send(` <html> <body> <div id="root"></div> </body> </html> `); }); app.listen(3000, () => { console.log('Server listening on port 3000'); });
Now we can create a file called index.js in the root of our project directory and write the code for our client-side application. This code will use the React library to render a simple component called App into the div with the id of root:
import React from 'react'; import ReactDOM from 'react-dom'; function App() { return ( <div> Hello, World! </div> ); } ReactDOM.render(<App />, document.getElementById('root'));
Finally, we can add a script to our package.json file to start the server and build the client-side application using a tool such as webpack:
{ "scripts": { "start": "webpack && node server.js" } }
Now we can start the server and build the client-side application by running the following command:
npm start
This will start the server, build the client-side application, and open a new web browser window showing the rendered HTML.
This is a very simple example of building a server-rendered application using React and Express, but it should give you a sense of the basic steps involved. In a real-world application, you would likely have many more components and a more complex server-side rendering function, and you would also need to set up a router to handle different URLs and a build system to transpile and bundle your code.
In conclusion, building a modern, server-rendered web application using a JavaScript framework such as React or Vue.js involves setting up a development environment and build system, writing reusable components, setting up a router, writing a server-side rendering function, and setting up a server to serve the generated HTML. By following these steps, you can build a web application that is optimized for search engines, works well on a wide range of devices, and can be easily built and deployed.
Recommended Posts
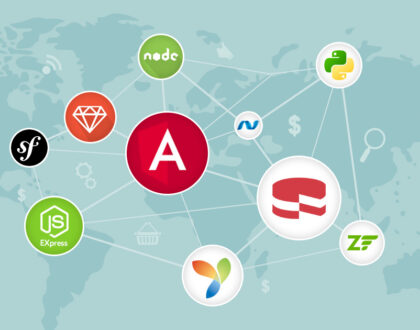
The most popular web development frameworks
December 11, 2022
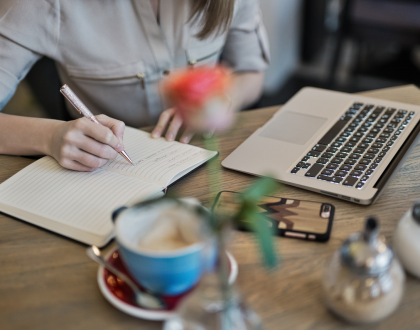
How To Create Content People Actually Care About
April 7, 2020
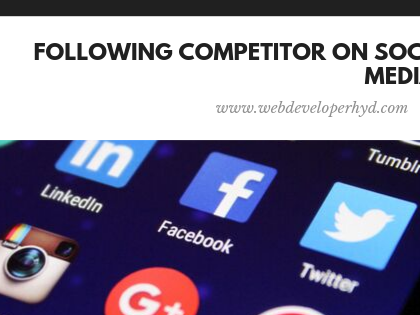
Follow Competitor on Social Media – 3 Best Reasons to do it!
October 7, 2019